1、注入TbScore的bean
1)、定义TbScore类
package com.shenmazong.pojo;
public class TbScore {
private Integer math;
private Integer history;
public TbScore(Integer math, Integer history) {
this.math = math;
this.history = history;
}
@Override
public String toString() {
return "TbScore{" +
"math=" + math +
", history=" + history +
'}';
}
}
2)、定义bean的注入
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="tbScore" class="com.shenmazong.pojo.TbScore">
<constructor-arg name="math" value="66"></constructor-arg>
<constructor-arg name="history" value="99"></constructor-arg>
</bean>
</beans>
3)、测试bean
package com.shenmazong;
import com.shenmazong.pojo.TbScore;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class DemoTest {
public static void main(String[] args) {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
TbScore tbScore = app.getBean(TbScore.class);
System.out.println(tbScore);
}
}
4)、运行项目
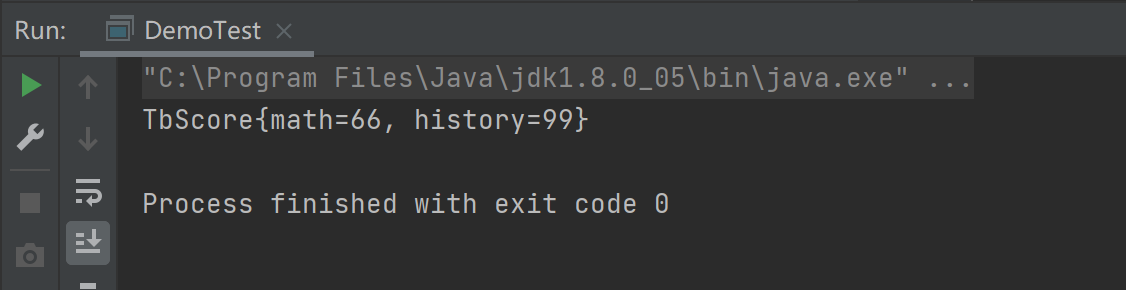
2)、set注入
1)、TbStudent类
package com.shenmazong.pojo;
public class TbStudent {
private String name;
private TbScore studentScore;
public TbStudent(String name) {
this.name = name;
}
public TbScore getStudentScore() {
return studentScore;
}
public void setStudentScore(TbScore studentScore) {
this.studentScore = studentScore;
}
@Override
public String toString() {
return "TbStudent{" +
"name='" + name + '\'' +
", studentScore=" + studentScore +
'}';
}
}
2)、注入配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="tbScore" class="com.shenmazong.pojo.TbScore">
<constructor-arg name="math" value="66"></constructor-arg>
<constructor-arg name="history" value="99"></constructor-arg>
</bean>
<bean id="tbStudent" class="com.shenmazong.pojo.TbStudent">
<constructor-arg name="name" value="武松"></constructor-arg>
<property name="studentScore" ref="tbScore"></property>
</bean>
</beans>
3)、测试代码
package com.shenmazong;
import com.shenmazong.pojo.TbScore;
import com.shenmazong.pojo.TbStudent;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class DemoTest {
public static void main(String[] args) {
ApplicationContext app = new ClassPathXmlApplicationContext("applicationContext.xml");
TbStudent tbStudent = app.getBean(TbStudent.class);
System.out.println(tbStudent);
}
}
4)、测试运行
